Python Libraries: Your Comprehensive Guide
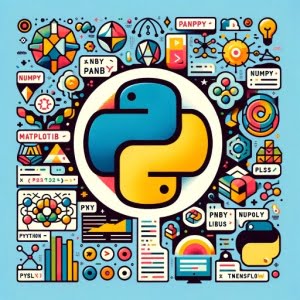
Ever felt overwhelmed by the vastness of Python’s capabilities? You’re not alone. Many developers find themselves at a crossroads when it comes to navigating the expansive world of Python. But, think of Python libraries as your personal guide – versatile and handy for various tasks.
In this guide, we’ll introduce you to Python libraries, their uses, and how to leverage them in your projects. We’ll cover everything from basic usage for beginners, to more advanced techniques for intermediate users, and even delve into creating your own libraries for the experts.
Let’s embark on this journey to master Python libraries!
TL;DR: What Are Python Libraries?
Python libraries are collections of reusable code modules that you can integrate into your projects to save time and effort. They are like a toolbox, each providing different tools that can be used to perform various tasks efficiently.
For instance, the math
library in Python provides a host of mathematical functions. Here’s a simple example:
import math
print(math.sqrt(16))
# Output:
# 4.0
In this example, we import the math
library and use its sqrt
function to calculate the square root of 16. The result, as expected, is 4.0.
This is just a glimpse of what Python libraries can do. There’s a whole universe of Python libraries waiting to be explored. Continue reading for a comprehensive guide on Python libraries, their uses, and how to leverage them in your projects.
Table of Contents
- Getting Started with Python Libraries
- Diving Deeper: Advanced Python Libraries
- Exploring Further: Creating and Using Third-Party Python Libraries
- Troubleshooting Common Issues with Python Libraries
- Understanding Python Libraries and Their Importance
- Python Libraries in Larger Projects
- Wrapping Up: Mastering Python Libraries
Getting Started with Python Libraries
Python libraries can seem daunting at first, but once you understand the basics, they become a powerful tool in your programming arsenal. Let’s start by understanding how to import and use a Python library with a simple example.
Importing and Using the math
Library
The math
library is a standard Python library that provides mathematical functions. To use it, we first need to import it into our program. Here’s how you do it:
import math
With the library imported, we can now use its functions. Let’s use the sqrt
function to calculate the square root of a number:
import math
print(math.sqrt(25))
# Output:
# 5.0
In this code, math.sqrt(25)
calls the sqrt
function from the math
library to calculate the square root of 25. The result is printed to the console.
This is a basic example of how to import and use a Python library. As you explore more libraries and their functions, you’ll see how they can simplify your code and make programming in Python much more efficient.
Popular Basic Functionality Python Libraries
Python has a rich and active ecosystem of libraries that provide a range of functionalities. Below is a table of some of the most popular Python libraries for basic functionality, along with their category and a brief description of each.
Library Name | Category | Description |
---|---|---|
math | Mathematics | Provides mathematical functions, including trigonometric, logarithmic, and other functions. |
datetime | Date and Time | Provides functions to manipulate dates and times, including time parsing, formatting, and arithmetic. |
os | Operating System | Provides a portable way of using operating system dependent functionality, like reading or writing to files. |
sys | System-specific | Allows Python programs to manipulate parts of the run-time environment such as command line arguments or exit status. |
random | Random Value | Generates pseudo-random numbers, enables random element selection, random permutations and more. |
json | JSON | Offers methods to manipulate JSON objects, including parsing, serializing, and deserializing. |
re | Regular Expressions | Provides functions to work with regular expressions for powerful string manipulation techniques. |
sqlite3 | Database | Allows interaction with SQLite database, a lightweight disk-based database that doesn’t require separate server process. |
csv | CSV Files | Allows the reading and writing of CSV files, which enables the manipulation and organization of data in table form. |
collections | Collections | Provides alternatives to built-in container data types such as list, set, dict, and tuple. Includes special types like Counter, deque and ordered dictionary. |
argparse | Command-line | Handles command-line options and arguments, and generates usage messages. Offers a straightforward way to obtain command-line arguments into your scripts. |
functools | Functional Programming | Provides tools for working with functions, such as higher-order functions, and functions that act on or return other functions. |
threading | Multithreading | Provides multiple threads of execution, useful when you want to improve the application performance by allowing multiple operations to run in parallel. |
multiprocessing | Multiprocessing | A package that supports spawning processes using an API similar to the threading module. |
urllib | URL handling modules | Fetch URLs and provides functionality for parsing URLs and changing between absolute and relative paths. |
statistics | Statistics | Provides functions to calculate mathematical statistics of numerical data. |
http.server | HTTP Servers | This module defines classes for implementing HTTP servers (Web servers). |
http.client | HTTP Clients | Contains classes for making HTTP requests. |
smtpd | SMTP Servers | Defines an SMTP server which can receive e-mails and optionally pass them on to a real SMTP server. |
smtplib | SMTP Client | The smtplib module defines an SMTP client session object that can be used to send mail to any Internet machine with an SMTP or ESMTP listener daemon. |
Diving Deeper: Advanced Python Libraries
As you gain confidence with Python libraries, you’ll discover their potential extends far beyond basic mathematical functions. Python libraries are instrumental in data analysis, web scraping, machine learning, and much more. Let’s explore some of these advanced uses.
Data Analysis with pandas
pandas
is a powerful Python library for data manipulation and analysis. It provides data structures and functions needed to manipulate structured data. Here’s an example of how to use pandas
to create a data frame from a dictionary and calculate the mean of the values:
import pandas as pd
# Create a dictionary
my_dict = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]}
# Convert the dictionary into a DataFrame
df = pd.DataFrame(my_dict)
# Calculate the mean age
mean_age = df['Age'].mean()
print(mean_age)
# Output:
# 30.0
In this example, we first import the pandas
library. We then create a dictionary and convert it into a pandas DataFrame. Finally, we calculate and print the mean age, which is 30.0.
Web Scraping with BeautifulSoup
BeautifulSoup
is a Python library used for web scraping purposes. It creates a parse tree from page source code that can be used to extract data in a hierarchical and more readable manner. Here’s a basic example of how to use BeautifulSoup
to scrape a website:
from bs4 import BeautifulSoup
import requests
# Make a request to the website
r = requests.get('http://www.example.com')
# Create an instance of the BeautifulSoup class to parse our webpage
soup = BeautifulSoup(r.text, 'html.parser')
# Use the 'find' method to find the first paragraph tag on the page
first_paragraph = soup.find('p')
print(first_paragraph.text)
# Output:
# 'This is an example paragraph.'
In this example, we first import the BeautifulSoup
and requests
libraries. We then make a request to a website and parse the HTML response using BeautifulSoup. Finally, we find and print the text of the first paragraph tag on the page.
These are just a few examples of how Python libraries can be used for more complex tasks. As you continue to explore, you’ll find that there’s a Python library for almost any task you can imagine!
Understanding Advanced Python Libraries
As we delve deeper into Python programming, we encounter libraries that provide more specialized and advanced functionality. These libraries are designed to tackle specific tasks and complex problems in fields like machine learning, natural language processing, network analysis, and others.
Below is a table of some of the more popular and common Python libraries with more advanced functionality:
Library Name | Category | Description |
---|---|---|
NumPy | Computation | A library for numerical computation, particularly useful for numerical matrix data. |
SciPy | Computing/Science | An open source Python library used for scientific, mathematical, and engineering computations. |
Pandas | Data | A data analysis and manipulation tool which provides flexible data structures. |
Matplotlib | Visualization | A plotting library that can produce line plots, bar graphs, histograms etc. |
Seaborn | Visualization | Provides a high-level interface for drawing attractive and informative statistical graphics. |
Bokeh | Visualization | Provides interactive plots and dashboards in modern web browsers for visualization. |
TensorFlow | Machine Learning/AI | An open-source library for machine learning and artificial intelligence. |
Keras | Machine Learning/AI | A high-level neural networks API, written in Python and capable of running on top of TensorFlow. |
PyTorch | Machine Learning/AI | Another open-source machine learning library based on the Torch library, used for applications such as computer vision and natural language processing. |
Scikit-learn | Machine Learning/AI | A library that provides simple and efficient tools for data mining and data analysis. |
NLTK | Natural Language Processing | Provides a practical introduction to programming for language processing. |
NetworkX | Networks | A package for the creation, manipulation, and study of the structure, dynamics, and functions of complex networks. |
Beautiful Soup | Web Scraping | Used for pulling data out of HTML and XML files. |
Requests | HTTP requests | A simple HTTP library for Python, built for human beings to interact with the internet. |
Pillow | Imaging | A fork of PIL (Python Image Library) that adds some user-friendly features. |
Pygame | Games | A suite of Python modules designed for writing games, but is also usable for creating other multimedia applications. |
SQLAlchemy | Database/ORM | The Python SQL toolkit and Object-Relational Mapping(ORM) library that gives application developers the full power and flexibility of SQL. |
Flask | Web Development | A micro web framework written in Python. It does not require particular tools or libraries. |
Django | Web Development | A high-level Python web framework that encourages rapid development and clean, pragmatic design. |
JupyterNotebook | Interactive Development Environment | An open-source web application that allows creation and sharing of documents that contain live code, equations, visualizations and narrative text. |
Exploring Further: Creating and Using Third-Party Python Libraries
As you progress in your Python journey, you might find the need to create your own libraries or use third-party libraries. These advanced techniques can provide even more flexibility and efficiency in your projects.
Modules from both the standard library and third party libraries need to be accessed via the import statement. Modules in the standard library, however, do not need to be separately installed in order to be able to import and use them.
Creating Your Own Python Library
Creating your own Python library can be a great way to organize and reuse your code. Here’s a simple example of how to create a Python library:
# my_library.py
def greet(name):
return f'Hello, {name}!'
In this code, we create a Python file my_library.py
and define a function greet
in it. This file can be imported as a library in other Python scripts.
# main.py
import my_library
print(my_library.greet('Alice'))
# Output:
# 'Hello, Alice!'
Here, we import the my_library
library in our main.py
script and use its greet
function. The output is ‘Hello, Alice!’.
Using Third-Party Python Libraries
Third-party Python libraries are libraries that are not part of the standard Python library. They are created by the community and can be used to perform a wide range of tasks. For example, requests
is a popular third-party library used for making HTTP requests:
import requests
response = requests.get('http://www.example.com')
print(response.status_code)
# Output:
# 200
In this example, we import the requests
library and use its get
function to make a GET request to a website. We then print the HTTP status code of the response, which is 200 indicating a successful request.
Whether you’re creating your own Python libraries or using third-party libraries, the key is to understand the problem you’re trying to solve and choose the right tool for the job. As you continue to explore Python libraries, you’ll find they can greatly enhance your productivity and the quality of your code.
Troubleshooting Common Issues with Python Libraries
Like any other programming tools, Python libraries can sometimes throw a curveball at you. Understanding common issues and their solutions can save you a lot of time and frustration. Let’s discuss some of these issues and how to resolve them.
Library Import Errors
One of the most common issues you might encounter is an import error. This usually happens when Python can’t find the library you’re trying to import. Here’s an example:
import non_existent_library
# Output:
# ImportError: No module named 'non_existent_library'
In this example, we’re trying to import a library that doesn’t exist, which results in an ImportError
. The solution is to ensure that the library you’re trying to import is installed and that you’ve spelled its name correctly.
Version Conflicts
Another common issue is version conflicts. Different projects might require different versions of the same library, which can lead to conflicts. Here’s an example of how to check the version of a Python library:
import pandas as pd
print(pd.__version__)
# Output:
# '1.2.4'
In this example, we import the pandas
library and print its version. If your project requires a different version, you can use a virtual environment to isolate your project and its dependencies.
These are just a couple of common issues you might encounter when working with Python libraries. Remember, every problem has a solution, and understanding these solutions is an important part of becoming a proficient Python programmer.
Understanding Python Libraries and Their Importance
Python libraries are a collection of reusable Python functions and methods bundled together. They’re like a toolbox, each tool serving a different purpose, making Python a versatile language for all kinds of programming tasks.
The Concept of Code Reuse
One of the fundamental principles of good programming is ‘Don’t Repeat Yourself’ (DRY). Reusing code helps maintain this principle, and Python libraries are a perfect example of this. Let’s look at a simple example using the math
library again:
import math
print(math.pi)
print(math.sqrt(16))
# Output:
# 3.141592653589793
# 4.0
In this example, we’re using the math
library to access the mathematical constant pi and calculate the square root of 16. This saves us from having to define these values or functions ourselves in our code, thus promoting code reuse.
Embracing Modular Programming
Modular programming is a design technique that separates the functionality of a program into independent, interchangeable modules. Python libraries are a form of modular programming. Each library is a module that can be imported and used in your code. This allows for better organization, easier debugging, and increased readability of your code.
Python libraries, with their wide array of functions and methods, are a testament to Python’s versatility. They allow for efficient and effective programming, promoting code reuse and modular programming. Whether you’re a beginner or an expert, understanding and utilizing Python libraries is a crucial step in mastering Python.
Python Libraries in Larger Projects
Python libraries are not just for small scripts or individual tasks. They play a fundamental role in larger projects, providing pre-built functionality, improving code readability, and reducing development time.
Python Modules, Packages, and Virtual Environments
As you delve deeper into Python, you’ll come across related topics like Python modules, packages, and virtual environments. A Python module is a .py file containing Python definitions and statements. A package is a way of organizing related modules into a directory hierarchy. Virtual environments, on the other hand, are a way to keep the dependencies required by different projects separate.
These concepts are closely related to Python libraries and further enhance the modularity and efficiency of Python programming.
Further Resources for Python Library Proficiency
To further your understanding of Python libraries, here are some resources that provide in-depth knowledge and practical examples:
- Python Import from Different Directory: A Quick Guide on how to import modules from different directories in Python.
Exploring Scientific Tools with SciPy in Python – Dive into SciPy’s extensive features for optimization, integration, and more.
Python’s Standard Library Documentation – The official documentation of Python’s standard library.
Python Module of the Week – A series of articles providing a tour of the Python standard library through short examples.
Awesome Python provides a curated list of awesome Python frameworks, libraries, and software.
Remember, mastering Python libraries is a journey. The more you practice and explore, the more proficient you’ll become. Happy coding!
Wrapping Up: Mastering Python Libraries
In this all-encompassing guide, we’ve journeyed through the dynamic world of Python libraries, exploring their vast capabilities and their pivotal role in Python programming.
We began with the basics, learning how to import and use standard Python libraries. We then ventured into more advanced territory, exploring complex tasks using Python libraries for data analysis and web scraping. We even delved into creating our own Python libraries and using third-party libraries, providing you with a wide range of tools for your Python projects.
Along the way, we tackled common challenges you might encounter when using Python libraries, such as import errors and version conflicts, providing you with solutions and workarounds for each issue.
We also looked at related concepts like Python modules, packages, and virtual environments, further enhancing your understanding of Python programming. Here’s a quick comparison of some popular Python libraries we discussed:
Library | Purpose | Pros | Cons |
---|---|---|---|
math | Mathematical functions | Easy to use, part of standard library | Limited functionality |
pandas | Data manipulation and analysis | Powerful, flexible | Can be memory-intensive |
BeautifulSoup | Web scraping | Easy to parse HTML and XML | Requires additional requests library |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your Python skills, we hope this guide has given you a deeper understanding of Python libraries and their capabilities. Happy coding!