Using ‘in’ with Python | Membership Operators Guide
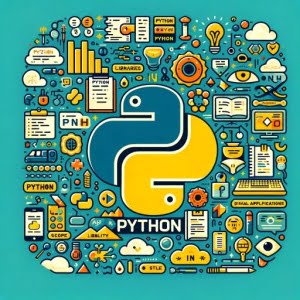
Ever found yourself in a situation where you needed to check if a value exists in a list, tuple, or string in Python? It’s a common requirement, and Python provides a simple yet powerful tool to accomplish this – the 'in'
operator. Think of it as a detective, tirelessly searching through your sequences to find what you’re looking for.
This guide will introduce you to the ‘in’ membership operator in Python and how to use it effectively. We’ll explore its basic usage, delve into more advanced scenarios, and even discuss alternative approaches.
So, let’s dive in and start mastering the ‘in’ operator in Python!
TL;DR: How Do I Use the ‘in’ Operator in Python?
The
'in'
operator in Python is used to check if a value exists in a sequence like a list, tuple, or string. EX:print(1 in list)
prints True if the value 1 is found inlist
, and False otherwise.
Here’s a simple example:
list = [1, 2, 3]
print(1 in list)
# Output:
# True
In this example, we have a list of numbers, and we’re using the ‘in’ operator to check if the number 1 is in this list. The operator returns True, indicating that 1 is indeed in the list.
This is just the tip of the iceberg when it comes to using the ‘in’ operator in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Basic Usage of the ‘in’ Operator in Python
- ‘in’ Operator with Nested Sequences and Dictionaries
- Exploring Alternatives to the ‘in’ Operator in Python
- Troubleshooting Common Issues with the ‘in’ Operator
- Python’s Sequences and the Concept of Membership
- The Relevance of the ‘in’ Operator in Python: Beyond the Basics
- Wrapping Up: Mastering the ‘in’ Operator in Python
Basic Usage of the ‘in’ Operator in Python
The ‘in’ operator in Python is straightforward to use. It checks whether a value exists in a sequence (such as a list, tuple, or string) and returns a Boolean value: True if the value is in the sequence, and False if it’s not.
Let’s see it in action with a list of numbers:
numbers = [1, 2, 3, 4, 5]
print(3 in numbers)
# Output:
# True
In this example, we’re checking if the number 3 is in our list of numbers. The ‘in’ operator returns True, indicating that 3 is indeed in the list.
The ‘in’ operator also works with strings. It can check if a substring exists within a larger string. Here’s an example:
sentence = 'The quick brown fox jumps over the lazy dog'
print('fox' in sentence)
# Output:
# True
In this case, we’re checking if the word ‘fox’ is in our sentence. Again, the ‘in’ operator returns True, indicating that ‘fox’ is part of the sentence.
The ‘in’ operator is a powerful tool in Python, but it’s not without its pitfalls. One common mistake is using it to compare numbers and strings. For example, the following code will not work as you might expect:
numbers = ['1', '2', '3', '4', '5']
print(1 in numbers)
# Output:
# False
In this example, the ‘in’ operator returns False, even though ‘1’ is in the list. That’s because it’s comparing the integer 1 with the string ‘1’, which are not the same. So, when using the ‘in’ operator, make sure the types of your value and the elements in your sequence match.
‘in’ Operator with Nested Sequences and Dictionaries
The ‘in’ operator is not just limited to simple sequences like lists or strings. It can also be used with more complex data structures such as nested sequences and dictionaries.
Nested Sequences
A nested sequence is a sequence that contains other sequences as its elements. For example, a list of lists is a nested sequence.
Let’s see how the ‘in’ operator works with nested sequences:
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
print([4, 5, 6] in nested_list)
# Output:
# True
In this example, we’re checking if the list [4, 5, 6]
is in our nested list. The ‘in’ operator returns True, indicating that [4, 5, 6]
is indeed a sublist of our nested list.
Dictionaries
The ‘in’ operator can also be used with dictionaries to check if a key is in the dictionary. Here’s an example:
person = {'name': 'Alice', 'age': 25, 'city': 'New York'}
print('age' in person)
# Output:
# True
In this case, we’re checking if the key ‘age’ is in our person dictionary. The ‘in’ operator returns True, indicating that ‘age’ is indeed a key in the dictionary.
Remember that the ‘in’ operator checks for keys in a dictionary, not values. If you want to check if a value is in the dictionary, you can use the values()
method:
print(25 in person.values())
# Output:
# True
In this example, the ‘in’ operator returns True, indicating that 25 is indeed a value in the dictionary.
Exploring Alternatives to the ‘in’ Operator in Python
The ‘in’ operator is a powerful tool for checking membership in Python, but it’s not the only tool available. There are other methods that can be used to achieve the same result, each with its own strengths and weaknesses. Let’s explore some of these alternatives.
Using the count()
Function
The count()
function can be used to check if a value exists in a sequence. It returns the number of times the value appears in the sequence. Here’s an example:
numbers = [1, 2, 3, 4, 5]
print(numbers.count(3) > 0)
# Output:
# True
In this example, we’re using the count()
function to check if the number 3 is in our list of numbers. The function returns the number of times 3 appears in the list, which we then check is greater than 0. The expression returns True, indicating that 3 is indeed in the list.
List Comprehension
List comprehension is another powerful feature of Python that can be used to check membership. Here’s an example:
numbers = [1, 2, 3, 4, 5]
print(any([True for num in numbers if num == 3]))
# Output:
# True
In this case, we’re using list comprehension to create a new list of Boolean values, where each value is True if the corresponding element in the numbers list is 3, and False otherwise. The any()
function then checks if any value in this new list is True.
While these methods can be useful in some scenarios, they’re generally not as efficient or readable as the ‘in’ operator. Therefore, it’s recommended to use the ‘in’ operator for checking membership in most cases. However, understanding these alternatives can help you write more flexible and efficient code when the situation calls for it.
Troubleshooting Common Issues with the ‘in’ Operator
While the ‘in’ operator is a powerful tool, like any feature in Python, it can present its own set of challenges. Let’s discuss some of the common issues you might encounter when using the ‘in’ operator, and how to troubleshoot them.
Type Errors
One common issue is type errors. These occur when you try to use the ‘in’ operator to compare incompatible types. For example, trying to check if an integer is in a list of strings will result in a False outcome, not a type error, but it might not be the result you’re expecting.
numbers = ['1', '2', '3']
print(1 in numbers)
# Output:
# False
In this example, the ‘in’ operator returns False, even though ‘1’ is in the list. That’s because it’s comparing the integer 1 with the string ‘1’, which are not the same.
To avoid this issue, make sure the types of your value and the elements in your sequence match.
Performance Issues with Large Sequences
The ‘in’ operator can be slow when used with large sequences. This is because it needs to check each element in the sequence until it finds a match.
If performance is a concern, and you’re working with a large sequence, consider using a set instead of a list or tuple. Sets in Python are implemented as hash tables, which provide constant time complexity for the ‘in’ operator, regardless of the size of the set.
Here’s an example:
large_list = list(range(1000000))
large_set = set(range(1000000))
# Using 'in' with a list
%timeit -n 10 999999 in large_list
# Using 'in' with a set
%timeit -n 10 999999 in large_set
In this example, you’ll find that using ‘in’ with a set is significantly faster than using it with a list.
Remember, the right tool for the job depends on the job. The ‘in’ operator is a powerful and flexible tool, but it’s not always the best tool for every situation. Always consider the nature of your data and the requirements of your task when choosing how to check membership in Python.
Python’s Sequences and the Concept of Membership
Before diving deeper into the ‘in’ operator, it’s crucial to understand the fundamentals of Python’s sequences and the concept of membership.
Understanding Python’s Sequences
In Python, a sequence is an ordered collection of items. Lists, tuples, and strings are examples of sequences. Each item in a sequence has a position (or index) starting from 0 for the first item, 1 for the second, and so on.
Here’s an example of a list, which is a type of sequence:
fruits = ['apple', 'banana', 'cherry']
print(fruits[0])
# Output:
# 'apple'
In this example, ‘apple’ is the first item in the list, so it has an index of 0.
The Concept of Membership
Membership is a fundamental concept in Python. It refers to whether a value is an item in a sequence. The ‘in’ operator is used to check membership. It returns True if a value is found in the sequence, and False otherwise.
Here’s an example:
fruits = ['apple', 'banana', 'cherry']
print('banana' in fruits)
# Output:
# True
In this example, ‘banana’ is an item in the fruits list, so the ‘in’ operator returns True.
By understanding Python’s sequences and the concept of membership, you can better understand how the ‘in’ operator works and how to use it effectively in your Python code.
The Relevance of the ‘in’ Operator in Python: Beyond the Basics
The ‘in’ operator in Python is more than a simple tool for checking membership in sequences. Its applications are wide and varied, making it a critical tool in many areas of Python programming.
Data Analysis with the ‘in’ Operator
In data analysis, the ‘in’ operator is often used to filter data. For instance, you might have a list of user IDs, and you want to filter a larger dataset to only include records associated with these user IDs. The ‘in’ operator makes this task simple and efficient.
Web Scraping and the ‘in’ Operator
In web scraping, the ‘in’ operator can be used to check if certain elements or attributes exist in the scraped data. This can help in cleaning and processing the data, ensuring that only relevant information is kept.
Exploring Related Concepts
The ‘in’ operator is just one tool in Python’s powerful toolkit. Other related concepts worth exploring include list comprehension and set operations.
- List comprehension is a concise way to create lists based on existing lists. It can be combined with the ‘in’ operator to create powerful one-liners.
Set operations like union, intersection, and difference can be used to perform complex membership checks. They can be a more efficient alternative to the ‘in’ operator when dealing with large datasets.
Further Resources for Mastering Python’s ‘in’ Operator
To deepen your understanding of the ‘in’ operator and its applications, here are some resources to explore:
- IOFlood’s Guide to Python Keywords – Grasp Python’s key concepts through its essential keywords.
Python Multiline Comment: Enhancing Code Readability – Learn how to create multiline comments in Python.
Python None: Understanding Null Values – Understand the significance of “None” in Python for absence of value.
Python’s Official Documentation – A guide to Python’s data structures, including sequences and set operations.
Real Python – An in-depth tutorial on Python operators, including the ‘in’ operator.
Python for Beginners – A beginner-friendly guide to list comprehension in Python.
Remember, the key to mastering any programming concept is practice. So, don’t just read about these concepts—try them out in your own Python projects!
Wrapping Up: Mastering the ‘in’ Operator in Python
In this comprehensive guide, we’ve journeyed through the world of the ‘in’ operator in Python, a powerful tool for checking membership in sequences.
We began with the basics, learning how to use the ‘in’ operator with simple sequences like lists, tuples, and strings. We then explored more advanced scenarios, such as using the ‘in’ operator with nested sequences and dictionaries.
Along the way, we tackled common challenges you might face when using the ‘in’ operator, such as type errors and performance issues with large sequences, providing you with solutions and workarounds for each issue.
We also explored alternative approaches to checking membership in Python, such as using the count()
function and list comprehension. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
‘in’ Operator | Simple, readable, works with all sequences | Can be slow with large sequences |
count() Function | Works with all sequences | Can be slow with large sequences |
List Comprehension | Flexible, can incorporate complex conditions | Can be hard to read, less efficient |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of the ‘in’ operator and its capabilities.
With its simplicity and versatility, the ‘in’ operator is a fundamental tool for Python programming. Happy coding!