Suppose that you have a C++ function or method that takes a string view as input:
void DoSomething(std::wstring_view sv)
{
// Do stuff ...
}
This function is invoked from some ATL or MFC code that uses the CString class. The code is built with Visual Studio C++ compiler in Unicode mode. So, CString is actually CStringW. And, to make things simpler, the matching std::wstring_view is used by DoSomething.
How can you pass a CString object to that function expecting a string view?
If you try directly passing a CString object like this:
CString s = L"Connie";
DoSomething(s); // *** Doesn't compile ***
you get a compile-time error. Basically, the C++ compiler complains about no suitable user-defined conversion from ATL::CString to std::wstring_view exists.
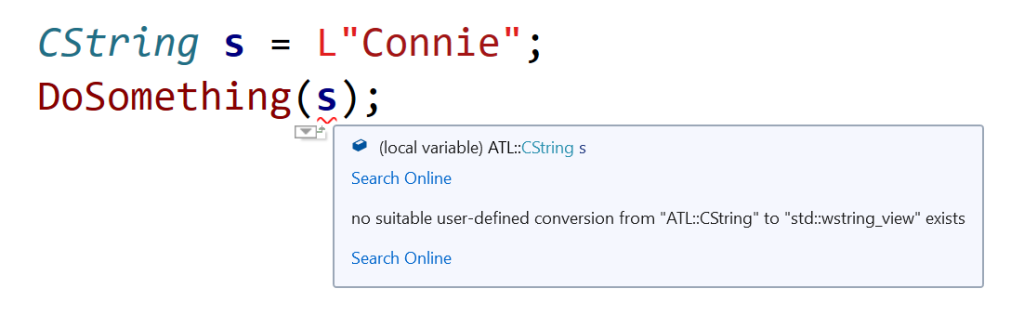
So, how can you fix that code?
Well, since there is a wstring_view constructor overload that creates a view from a null-terminated character string pointer, you can invoke the CString::GetString method, and pass the returned pointer to the DoSomething function expecting a string view parameter, like this:
// Pass the CString object 's' to DoSomething
// as a std::wstring_view
DoSomething(s.GetString());
Now the code compiles correctly!
Important Note About String Views
Note that wstring_view is just a view to a string, so you must pay attention that the pointed-to string is valid for at least all the time you are referencing it via the string view. In other words, pay attention to dangling references and string views that refer to strings that have been deallocated or moved elsewhere in memory.
One thought on “Adventures with C++ string_view: Interop with ATL/MFC CString”